Generating synthetic images that have values between 0 and 1 are very useful for imaging. It can serve as a cropping tool, a noise lessening tool, an aperture and other various uses for image processing. Five images were generated in this exercise, a square, a sinusoid, a grating, an annulus, and a Gaussian graded circle.
First, let us see the generated square image. The square is made on a space matrix spanning from -100 to 100 arbitrary units (a.u.) for both the x and y axis. The code used to generate this is shown below,
nx = 300; ny = 300; // sets the number of data points per axis
x = linspace(-100,100,nx); // creates an axis that span from -100 to 100 a.u.
y = linspace(-100,100,ny); // creates an axis that span from -100 to 100 a.u.
[X,Y] = ndgrid(x,y); // creates a space matrix using the generated axis
Squa = ones(nx,ny); // creates an image space
Squa(find(abs(X)>70)) = 0; // creates the square image
Squa(find(abs(Y)>70)) = 0; // creates the square image
imwrite(Squa,'Square.jpg'); // saves the image into a JPEG file
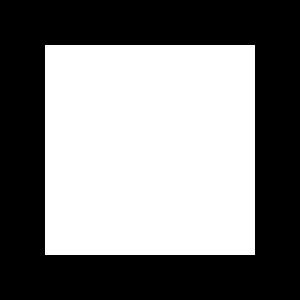
Figure 1. Generated Square image having sides equal to 70 a.u.
Figure 1 can serve many purpose for image processing, it can be an aperture or a tool that crops images to desired size.
The second image is a sinusoid propagating through the x axis. Again the sinusoid is created on the same space matrix used in the square image. The period of the sinusoid on the x axis is 25 a.u. and the code used to generate it is shown below,
nx = 300; ny = 300; // sets the number of data points per axis
x = linspace(-100,100,nx); // creates an axis that span from -100 to 100 a.u.
y = linspace(-100,100,ny); // creates an axis that span from -100 to 100 a.u.
[X,Y] = ndgrid(x,y); // creates a space matrix using the generated axis
Sine_func = (1+sin(2*%pi*Y/25))/2; // generates the sinusoidal image with a period of 25 a.u
imwrite(sine_func,'Sinusoid.jpg'); // saves the image into JPEG file
Figure 2. Generated Sinusoidal image having a period of 25 a.u.
The image on figure 2 can serve as an aperture for image processing. Also, I personally think that it can lessen noise that is horizontally periodic in an image.
The next image is a grating along the x axis. This image is different from the previously generated images because the grating is generated on a space matrix spanning from -150 to 149 a.u. with interval steps for both the x axis and y axis. The image was generated using the code below,
nx = 300; ny = 300; // sets the number of data points per axis
x = [-150:1.0:149]; // creates an axis from -150 to 149 a.u. with interval steps
y = [-150:1.0:149]; // creates an axis from -150 to 149 a.u. with interval steps
[X,Y] = ndgrid(x,y); // creates the space matrix using the generated axis
Grat = ones(nx,ny); // creates the image matrix
Grat(find(pmodulo(Y,3)==0)) = 0; // generates the grating image with grating size of 3 a.u.
imwrite(Grat,'Grating.jpg'); // saves the image into a JPEG file
Figure 3. Generated Grating image with grating size of 3 a.u.
The image in figure 3 is very useful for simulating grating effects on images. By adjusting the grating size, various diffraction effects can be observed.
The fourth image is an annular having inner diameter, ri, and outer diameter, ro. This image is generated in the same space matrix used for the square and sinusoid image. The code used is shown below,
nx = 300; ny = 300; // sets the number of data points per axis
x = linspace(-100,100,nx); // creates an axis that span from -100 to 100 a.u.
y = linspace(-100,100,ny); // creates an axis that span from -100 to 100 a.u.
[X,Y] = ndgrid(x,y); // creates a space matrix using the generated axis
Annu = zeros(nx,ny); // creates the image matrix
r = X.^2 + Y.^2; // converts cartessian coordinates into cylindrical coordinates
Annu(find(r<80))>= 1; // creates the outer circle
Annu(find(r<60)) = 0; // creates the inner circle
imwrite(Annu,'Annulus.jpg'); // saves the image into a JPEG file
Figure 4. Generated Annulus image having an outer radius of 80 a.u and an inner radius of 60 a.u.
The annulus on figure 4 can be used as an aperture that can serve as a bandpass filter in Fourier space.
The last image generated is a circle with a gradient transparency (Gaussian transparency). Again, this is generated in the same space matrix used for the square image. The code used in generating this image is shown below,
nx = 300; ny = 300; // sets the number of data points per axis
x = linspace(-100,100,nx); // creates an axis that span from -100 to 100 a.u.
y = linspace(-100,100,ny); // creates an axis that span from -100 to 100 a.u.
[X,Y] = ndgrid(x,y); // creates a space matrix using the generated axis
Gau = exp(-(X.^2+Y.^2)/(50)^2); // generates the circle with Gaussian transparency
imwrite(Gau,'Gaussian.jpg'); // saves the image into a JPEG file
Figure 5. Generated Gaussian image with λ = √(50 a.u.)
At first I thought that this generated Gaussian image is the circular aperture with Gaussian transparency, but because of my colleague Androphil Polinar, I realized that there is still a part missing with my code and the generated image.
So now the code looks like this,
nx = 300; ny = 300; // sets the number of data points per axis
x = linspace(-100,100,nx); // creates an axis that span from -100 to 100 a.u.
y = linspace(-100,100,ny); // creates an axis that span from -100 to 100 a.u.
[X,Y] = ndgrid(x,y); // creates a space matrix using the generated axis
Gau = exp(-(X.^2+Y.^2)/(50)^2); // generates Gaussian transparency
Gau(find(X.^2 + Y.^2>60)) = 0; // creates the circular aperture
imwrite(Gau,'Gaussian.jpg'); // saves the image into a JPEG file
Figure 6. Generated Circular aperture with Gaussian gradient image with radius 60 a.u. and λ = √(50 a.u.)
The Gaussian transparent circular aperture can greatly be of used in sharpening an image. It will be a great kernel in a convolution process to sharpen centered images in image processing.
The images that were generated here are only a few of the many synthetic images that can be generated using Scilab. The user’s imagination and creativity is the only limitation.
In this activity, I would give myself a 10 not only because I had fun, but I also learned a lot of techniques in generating synthetic images in Scilab. =)
Reference:
Dr. Soriano. Applied Physics 186 activity handouts: A2 - Scilab Basics
No comments:
Post a Comment